Make An HTML Version of the iPhone's Contact Screen.
We want this, but not so blurry.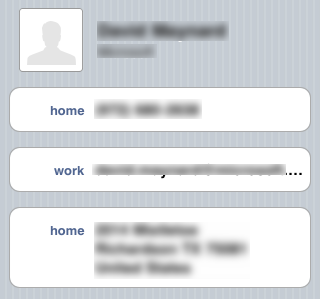
I'm going to break it down with a heading, text and a list. I'll go with a definition list. We define the phone numbers, email and address.
Let's mark up the base.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Person</title>
<!--[if IE]>
<script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
<style>
article, aside, details, figcaption, figure, footer, header,
hgroup, menu, nav, section { display: block; }
</style>
</head>
<body>
<h2>John Ivanoff</h2>
<p>Some Company</p>
<dl>
<dt>work</dt>
<dd>972-555-1212</dd>
<dt>home</dt>
<dd>972-555-1212</dd>
</dl>
<dl>
<dt>work</dt>
<dd><a href="mailto:unknown@unknown.org">unknown@unknown.org</a></dd>
</dl>
<dl>
<dt>work</dt>
<dd><a href="http://goo.gl/1nTU8">123 Chicago Ave<br/>Lebanon, KS 76051-7693 </a></dd>
</dl>
</body>
</html>
View it in a browser and we get.<html>
<head>
<meta charset="utf-8" />
<title>Person</title>
<!--[if IE]>
<script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
<style>
article, aside, details, figcaption, figure, footer, header,
hgroup, menu, nav, section { display: block; }
</style>
</head>
<body>
<h2>John Ivanoff</h2>
<p>Some Company</p>
<dl>
<dt>work</dt>
<dd>972-555-1212</dd>
<dt>home</dt>
<dd>972-555-1212</dd>
</dl>
<dl>
<dt>work</dt>
<dd><a href="mailto:unknown@unknown.org">unknown@unknown.org</a></dd>
</dl>
<dl>
<dt>work</dt>
<dd><a href="http://goo.gl/1nTU8">123 Chicago Ave<br/>Lebanon, KS 76051-7693 </a></dd>
</dl>
</body>
</html>
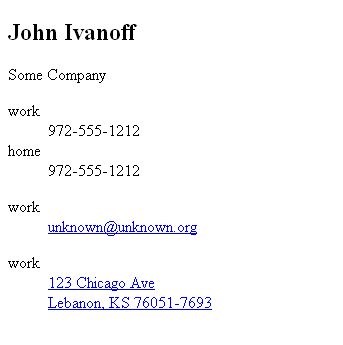
We have the foundation. Now let's add some style.
We'll start with the background of the page.
body {
background-color: #ddd; /* Background color */
color: #222; /* Foreground color used for text */
font-family: Helvetica;
font-size: 14px;
margin: 0; /* Amount of negative space around the outside of the body */
padding: 0; /* Amount of negative space around the inside of the body */
}
View it in a browser and we get.background-color: #ddd; /* Background color */
color: #222; /* Foreground color used for text */
font-family: Helvetica;
font-size: 14px;
margin: 0; /* Amount of negative space around the outside of the body */
padding: 0; /* Amount of negative space around the inside of the body */
}
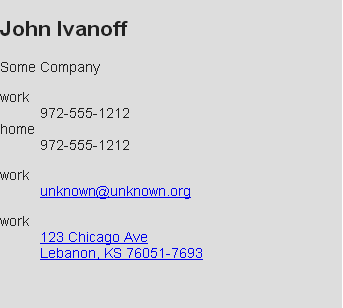
Let's get the lists styled.
We'll add the white background and border.
dl {
list-style: none;
padding: 0;
}
dt, dd {
background-color: #fff; /* Background color */
color: #222222; /* Foreground color used for text */
border: 1px solid #999;
font-weight: bold;
margin: 0;
padding: 12px 10px;
}
dt:first-child {
-webkit-border-top-left-radius: 8px;
}
dt:first-child + dd { /* This rounds the first definition item */
-webkit-border-top-left-radius: 8px;
-webkit-border-top-right-radius: 8px;
}
dd:last-child {
-webkit-border-bottom-right-radius: 8px;
-webkit-border-bottom-left-radius: 8px;
}
View it in a browser.list-style: none;
padding: 0;
}
dt, dd {
background-color: #fff; /* Background color */
color: #222222; /* Foreground color used for text */
border: 1px solid #999;
font-weight: bold;
margin: 0;
padding: 12px 10px;
}
dt:first-child {
-webkit-border-top-left-radius: 8px;
}
dt:first-child + dd { /* This rounds the first definition item */
-webkit-border-top-left-radius: 8px;
-webkit-border-top-right-radius: 8px;
}
dd:last-child {
-webkit-border-bottom-right-radius: 8px;
-webkit-border-bottom-left-radius: 8px;
}
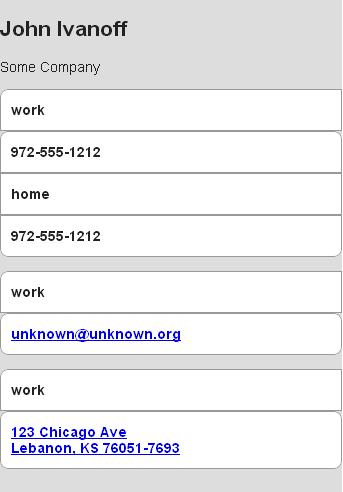
We'll style the 'terms.' We'll make them 60 pixels wide, have the text align to the right and make it a little lighter in color.
dt {
color: #516691;
text-align: right;
width: 60px;
}
View it in a browser.color: #516691;
text-align: right;
width: 60px;
}
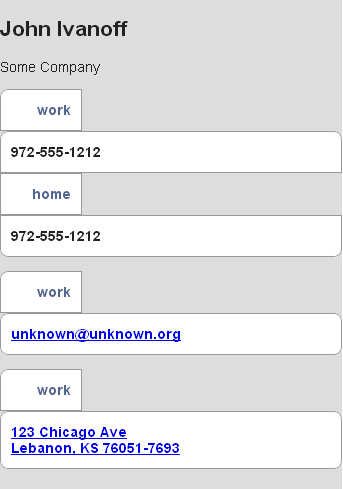
Now we'll move up the 'definitions' so it looks like one line. We'll float the dt
dt {
float: left; /* this will 'bring up' the dd */
color: #516691;
text-align: right;
width: 60px;
}
View it in a browser.float: left; /* this will 'bring up' the dd */
color: #516691;
text-align: right;
width: 60px;
}
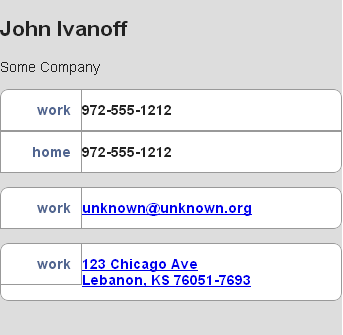
That doesn't look good. We'll remove the border on the dt and I'm picky here but I want to move the dt a little bit.
dt {
float: left; /* this will 'bring up' the dd */
border: 0; /* remove border */
padding-top: 13px; /* alignment tweaking */
color: #516691;
text-align: right;
width: 60px;
}
View it in a browser.float: left; /* this will 'bring up' the dd */
border: 0; /* remove border */
padding-top: 13px; /* alignment tweaking */
color: #516691;
text-align: right;
width: 60px;
}
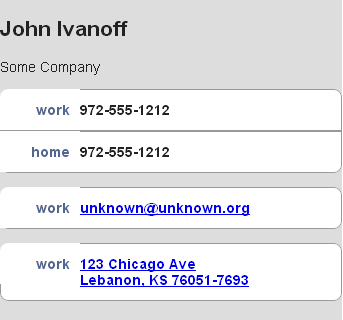
That's a little better but the top left looks like crud. The remedy? Make the color of the dt transparent.
dt {
float: left; /* this will 'bring up' the dd */
border: 0; /* remove border */
padding-top: 13px; /* alignment tweaking */
color: #516691;
text-align: right;
width: 60px;
background-color: transparent; /* clears up the top left corner */
}
View it in a browser. Bam!float: left; /* this will 'bring up' the dd */
border: 0; /* remove border */
padding-top: 13px; /* alignment tweaking */
color: #516691;
text-align: right;
width: 60px;
background-color: transparent; /* clears up the top left corner */
}
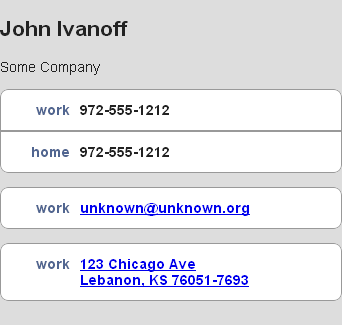
Looking good.
Let's work on the name and company. We'll wrap a div around the contact name and company
.......
<div id="namecompany">
<h2>John Ivanoff</h2>
<p>Some Company</p>
</div>
.....
We'll add this image like the on for a contact without a photo<div id="namecompany">
<h2>John Ivanoff</h2>
<p>Some Company</p>
</div>
.....

div#namecompany{
padding-left: 90px; /* Makes room for the photo */
background: transparent url(../i/person-icon.png) 10px 0 no-repeat; /* This sets up the position of the image */
height: 70px; /* Set the height of the div */
}
div#namecompany h2, div#namecompany p {
margin: 0; /* Just smash it all together */
}
View it in a browser.padding-left: 90px; /* Makes room for the photo */
background: transparent url(../i/person-icon.png) 10px 0 no-repeat; /* This sets up the position of the image */
height: 70px; /* Set the height of the div */
}
div#namecompany h2, div#namecompany p {
margin: 0; /* Just smash it all together */
}
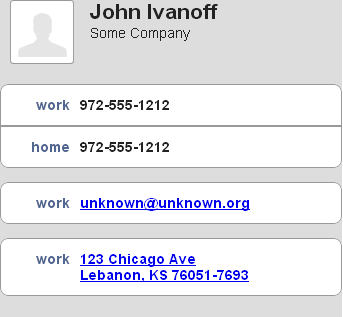
We're starting to cook with gas. A few minor areas to touch up. We need padding around the page.
We can hack it and add a margin to the entire page. The problem with that is when we add a header or footer that will ruin the look. The header or footer will not span the width of the screen.
What about wrapping everything in a div content? That add unnecessary HTML.
For this simple layout I'll add the padding to the namecompany div and the lists
div#namecompany, dl {
margin: 10px;
}
View it in a browser.margin: 10px;
}
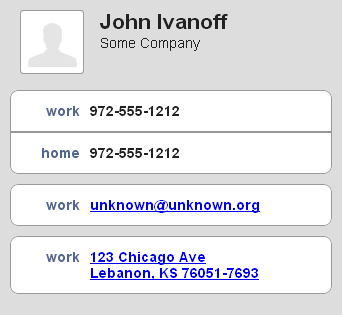
Smashing. Be'll make the phone numbers into links so we can call
...
<dl>
<dt>work</dt>
<dd><a href="tel:972-555-1212">972-555-1212</a></dd>
<dt>home</dt>
<dd><a href="tel:972-555-1213">972-555-1213</a></dd>
</dl>
...
View it in a browser.<dl>
<dt>work</dt>
<dd><a href="tel:972-555-1212">972-555-1212</a></dd>
<dt>home</dt>
<dd><a href="tel:972-555-1213">972-555-1213</a></dd>
</dl>
...
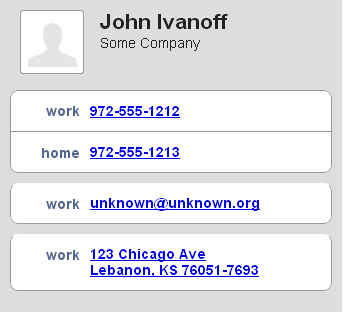
Let's hide those ugly links.
a {
text-decoration: none;
color: #000;
}
View it in a browser.text-decoration: none;
color: #000;
}
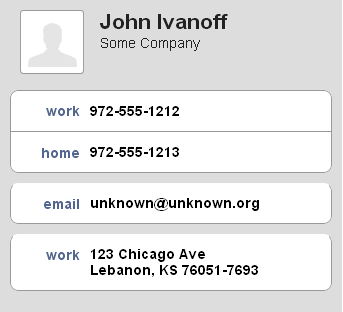
With this current design if you have a really long email the email will wrap just like the address. In the contacts app on the iPhone long emails are truncated with a trailing ellipsis.
We'll add a home email and make it a really long email address and we'll add a class of email to the dd
...
<dl>
<dt>work</dt>
<dd class="email"><a href="mailto:unknown@unknown.org">unknown@unknown.org</a></dd>
<dt>home</dt>
<dd class="email"><a href="mailto:unknown.unknown@areallyreallyreallylongurl.org"> unknown.unknown@areallyreallyreallylongurl.org</a></dd> </dl>
...
View it in a browser.<dl>
<dt>work</dt>
<dd class="email"><a href="mailto:unknown@unknown.org">unknown@unknown.org</a></dd>
<dt>home</dt>
<dd class="email"><a href="mailto:unknown.unknown@areallyreallyreallylongurl.org"> unknown.unknown@areallyreallyreallylongurl.org</a></dd> </dl>
...
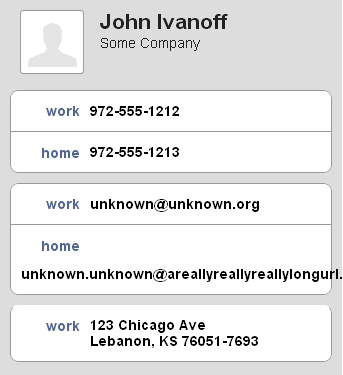
That's not good.
Let's add an ellipsis to text that is too long for its container.
.email {
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
}
View it in a browser.overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
}
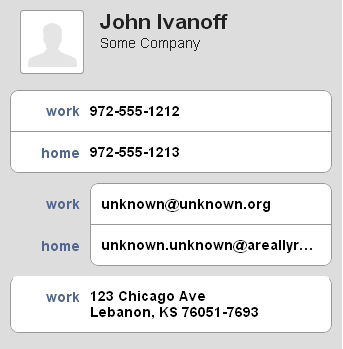
Still not good.
.email {
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
display: block;
width: inherit;
}
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
display: block;
width: inherit;
}
Update…
That code will render like the previous image.Move the .email class into the a element. The a element should look like the following
a {
text-decoration: none;
color: #000;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
display: block;
width: inherit;
}
text-decoration: none;
color: #000;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
display: block;
width: inherit;
}
Then remove the .email class from the <dd> for the emails,
Moving that code to the a element will also insure that any long text will be shortened to stay within the page.
View it in a browser.
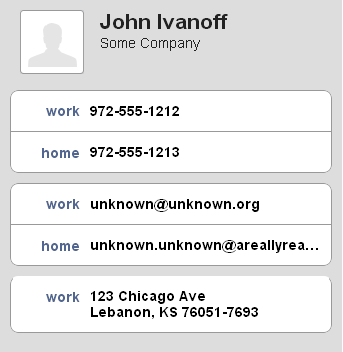
Hurray!
Let's add some finishing touches.
A title bar and the pinstriped background. Add this to the html code.
...
<h1>Info</h1>
...
Add to CSS code.<h1>Info</h1>
...
body {
background: #ddd url(../i/pinstripes.png) repeat scroll 0%;
color: #222; /* Foreground color used for text */
font-family: Helvetica;
font-size: 14px;
margin: 0; /* Amount of negative space around the outside of the body */
padding: 0; /* Amount of negative space around the inside of the body */
}
...
h1 {
color: #222;
font-size: 20px;
font-weight: bold;
margin: 0 auto;
padding: 10px 0;
text-align: center;
text-shadow: 0px 1px 0px #fff;
background-image: -webkit-gradient(linear, left top, left bottom,
from(#ccc), to(#999));
}
View it in a browser.background: #ddd url(../i/pinstripes.png) repeat scroll 0%;
color: #222; /* Foreground color used for text */
font-family: Helvetica;
font-size: 14px;
margin: 0; /* Amount of negative space around the outside of the body */
padding: 0; /* Amount of negative space around the inside of the body */
}
...
h1 {
color: #222;
font-size: 20px;
font-weight: bold;
margin: 0 auto;
padding: 10px 0;
text-align: center;
text-shadow: 0px 1px 0px #fff;
background-image: -webkit-gradient(linear, left top, left bottom,
from(#ccc), to(#999));
}
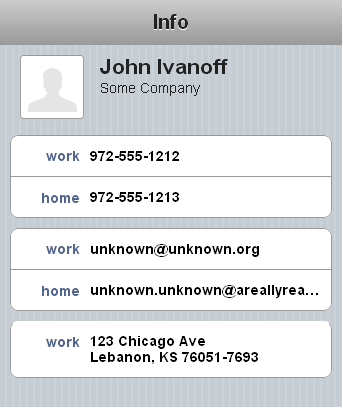
There we go, a web page that looks like a native iPhone app.